Clean and efficient code is essential for a fast, user-friendly website. While jQuery simplifies JavaScript development, improper usage can lead to bloated and slow-performing code. This article provides essential tips to write cleaner and faster jQuery code in 2025, helping you optimize your projects and improve user experience.
Why Optimize Your jQuery Code?
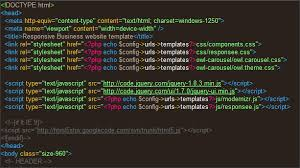
Optimizing jQuery code benefits your website by:
- Improving Page Speed: Faster websites rank higher in search engines and enhance user satisfaction.
- Reducing Bugs: Clean, well-structured code is easier to debug and maintain.
- Enhancing Scalability: Efficient code performs better as your website grows.
Essential jQuery Tips for Cleaner and Faster Code
1. Use the Latest jQuery Version
Always use the most up-to-date version of jQuery to benefit from performance improvements and security fixes. You can load it from a Content Delivery Network (CDN):
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
2. Minimize DOM Traversals
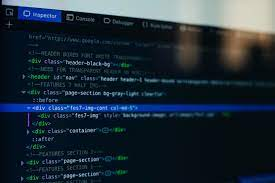
Accessing DOM elements repeatedly can slow down your code. Cache frequently accessed elements to avoid redundant lookups:
// Inefficient
$('.button').click(() => {
$('.button').addClass('active');
});
// Optimized
const $button = $('.button');
$button.click(() => {
$button.addClass('active');
});
3. Use Specific Selectors
Avoid using overly broad selectors like '*'
or .class
. Specific selectors improve performance and clarity:
// Avoid
$('div').addClass('highlight');
// Better
$('.content-box').addClass('highlight');
4. Leverage Event Delegation
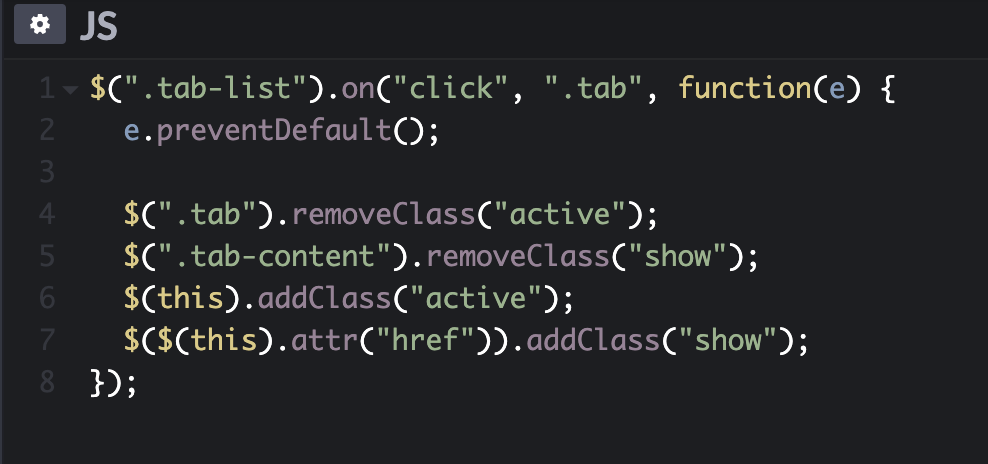
Instead of attaching events to multiple elements, use event delegation to manage events on a parent element. This reduces memory usage and simplifies event handling:
// Inefficient
$('li').click(() => alert('Item clicked!'));
// Optimized
$('ul').on('click', 'li', () => alert('Item clicked!'));
5. Remove Unused jQuery Plugins
Too many plugins can bloat your project. Regularly review your code to remove unused or outdated plugins. Choose lightweight alternatives when possible.
6. Optimize Animations
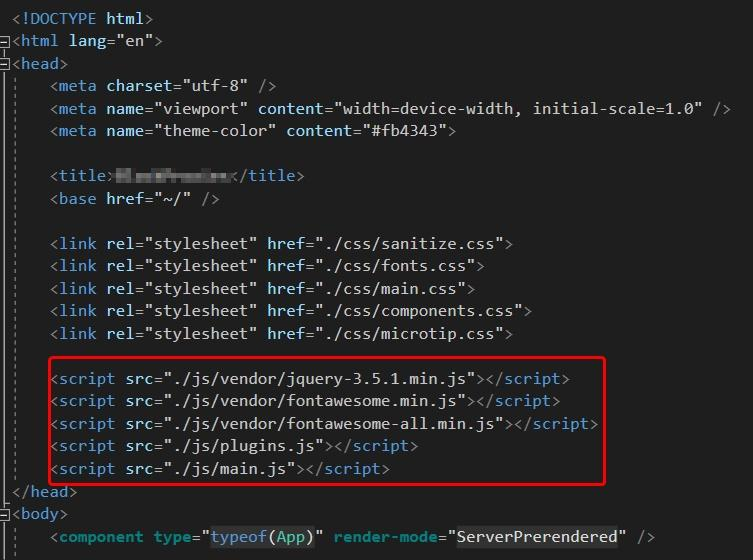
Animations can be resource-intensive. Use CSS animations where possible, and avoid chaining too many animation calls in jQuery:
// Avoid
$('.box').fadeOut(500).fadeIn(500).fadeOut(500);
// Better
$('.box').fadeOut(500, () => $(this).fadeIn(500));
7. Combine and Minify Scripts

Combine your JavaScript files into a single file and minify them to reduce file size and loading times. Tools like Webpack or Gulp can help automate this process.
8. Use $.fn
to Extend jQuery
If you find yourself reusing similar code, consider creating a custom jQuery plugin. This keeps your code DRY (Don’t Repeat Yourself):
// Custom Plugin
$.fn.changeColor = function(color) {
return this.css('color', color);
};
// Usage
$('p').changeColor('blue');
Remove event listeners when they’re no longer needed to free up resources:
// Attach Event
const handler = () => alert('Clicked!');
$('#myButton').on('click', handler);
// Detach Event
$('#myButton').off('click', handler);
10. Use Native JavaScript for Simple Tasks
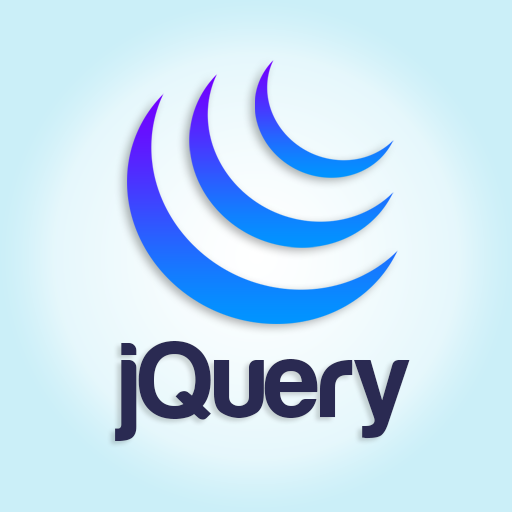
Modern JavaScript provides many features that eliminate the need for jQuery in some cases. For simple tasks, vanilla JavaScript can be faster:
// jQuery
$('#element').hide();
// Vanilla JavaScript
document.getElementById('element').style.display = 'none';
Conclusion
jQuery remains a powerful tool for web development in 2025, but writing clean and optimized code is key to getting the most out of it. By following these tips, you’ll create faster, more maintainable websites that deliver a better experience for users.
Start implementing these best practices today to streamline your jQuery code and boost your website’s performance!