Introduction
MongoDB is a powerful NoSQL database that allows developers to store and manage data efficiently. Knowing how to use essential MongoDB queries can help you retrieve, update, and manipulate data effectively. In this blog, we’ll explore five must-know MongoDB queries that every developer should be familiar with in 2025.
1. Finding Data with the Find Query
The find()
query is used to retrieve documents from a collection. It allows you to filter results based on specific conditions.
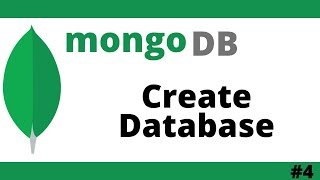
Example: Find all users from the users
collection.
// Retrieve all users
db.users.find({})
Example: Find users with age greater than 25.
// Retrieve users where age is greater than 25
db.users.find({ age: { $gt: 25 } })
2. Inserting Data with the Insert Query
The insertOne()
and insertMany()
methods allow you to add new documents to a collection.
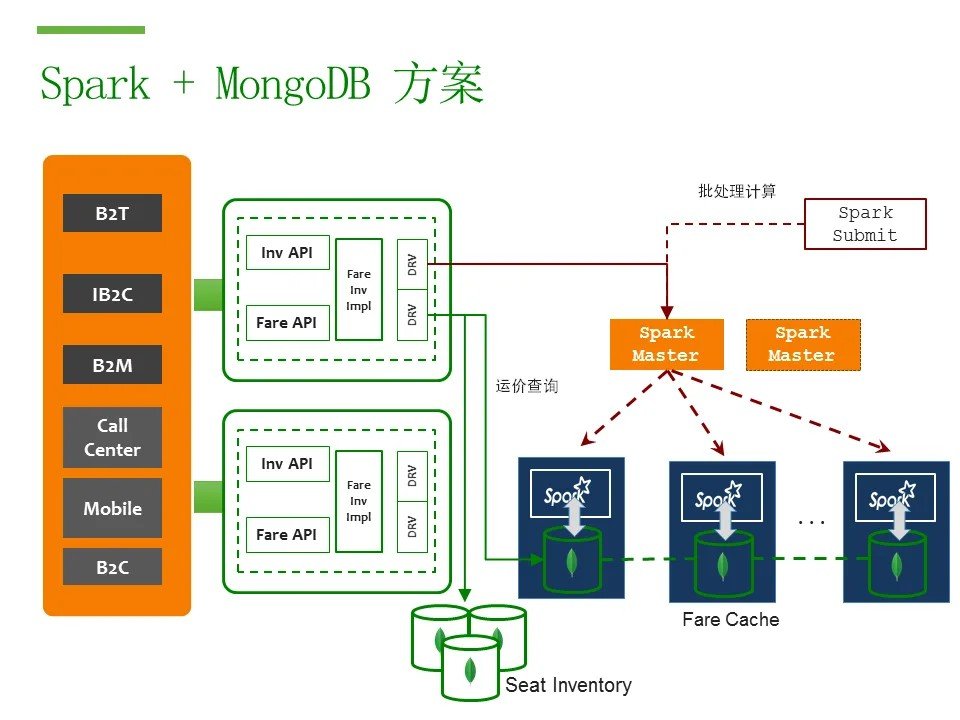
Example: Insert a new user into the users
collection.
// Insert a single user
db.users.insertOne({ name: "John Doe", age: 30, email: "john@example.com" })
Example: Insert multiple users at once.
// Insert multiple users
db.users.insertMany([
{ name: "Alice", age: 28, email: "alice@example.com" },
{ name: "Bob", age: 35, email: "bob@example.com" }
])
3. Updating Data with the Update Query
The updateOne()
and updateMany()
methods help modify existing documents in a collection.
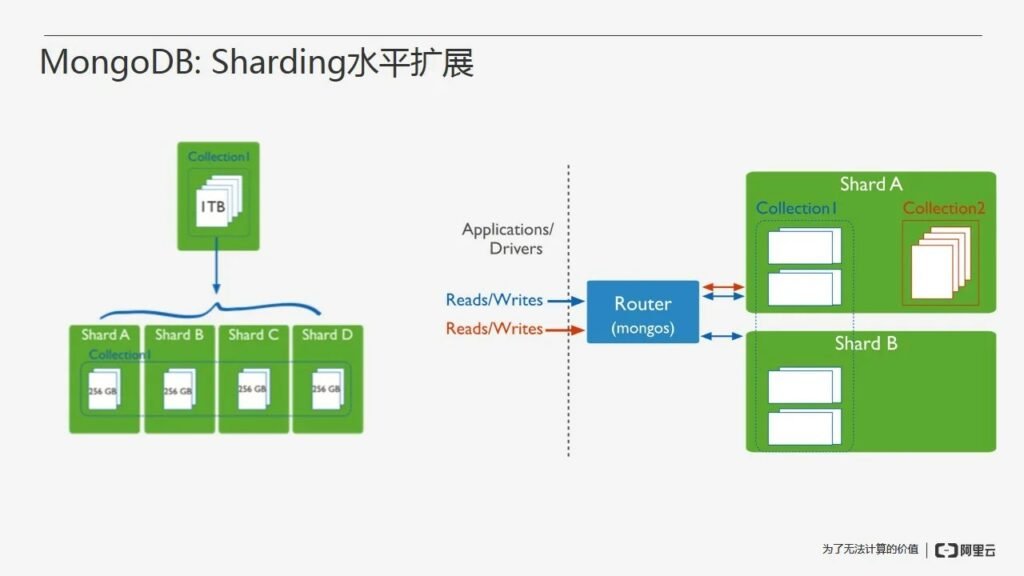
Example: Update a user’s email.
// Update email for a specific user
db.users.updateOne({ name: "John Doe" }, { $set: { email: "john.doe@example.com" } })
Example: Increase the age of all users by 1 year.
// Increment age for all users
db.users.updateMany({}, { $inc: { age: 1 } })
4. Deleting Data with the Delete Query
The deleteOne()
and deleteMany()
methods allow you to remove documents from a collection.
Example: Delete a specific user.
// Remove a user by name
db.users.deleteOne({ name: "Alice" })
Example: Delete all users older than 60.
// Remove all users where age is greater than 60
db.users.deleteMany({ age: { $gt: 60 } })
5. Aggregating Data with the Aggregate Query

The aggregate()
method is used to perform advanced data analysis and transformations.
Example: Group users by age and count how many users fall into each age group.
// Count users by age
db.users.aggregate([
{ $group: { _id: "$age", count: { $sum: 1 } } }
])
Example: Find the average age of all users.
// Calculate the average age
db.users.aggregate([
{ $group: { _id: null, averageAge: { $avg: "$age" } } }
])
Conclusion
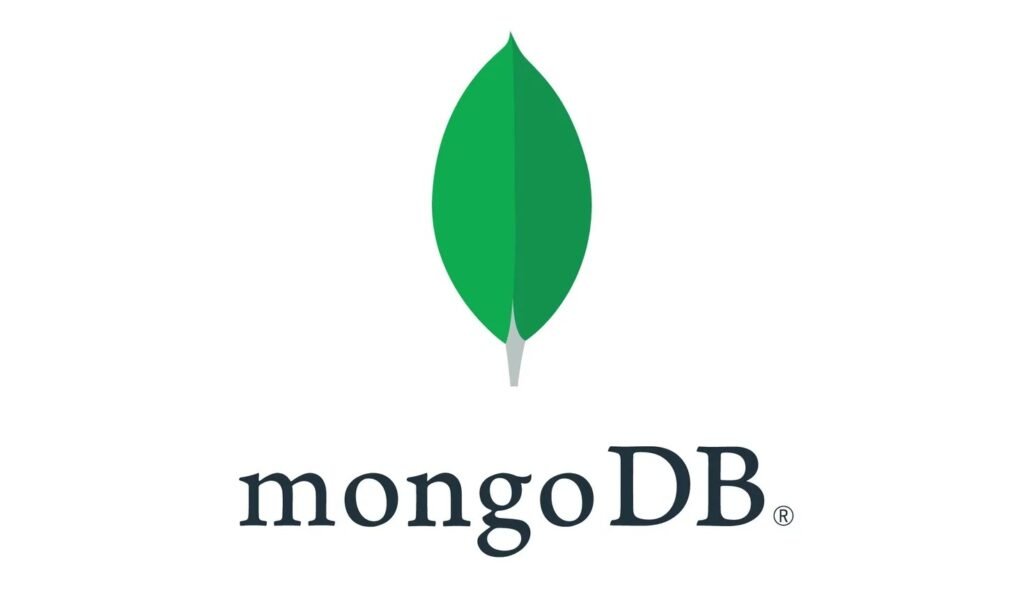
Mastering these essential MongoDB queries will help you work efficiently with data in your applications. Whether you’re retrieving, inserting, updating, deleting, or aggregating data, these queries provide a solid foundation for working with MongoDB.
Are you ready to enhance your MongoDB skills? Start practicing these queries today!
Keywords: MongoDB queries, MongoDB find, MongoDB insert, MongoDB update, MongoDB delete, MongoDB aggregate, MongoDB tutorial, MongoDB 2025, MongoDB best practices